Graphics And For Loops in Python Turtle
Graphics functions and loops in Python Turtle just go together! There's so much you can design in Python Turtle when you add a for loop to control the basic graphics functions. Share on XSteps to Drawing a Square
Think about the steps needed to draw a square:

The first thing you need to do is decide how long the sides will be. Since we’re using a computer screen, this measurement is in pixels. Now, write the steps down in order – as if you were drawing the square on paper:
- Move the turtle forward by x pixels.
- Turn left.
- Move the turtle forward by x pixels.
- Turn left.
- Move the turtle forward by x pixels.
- Turn left.
- Move the turtle forward by x pixels.
- Turn left.
Graphics And Loops Make Sense!
Look at the repetition in this code:

The first two steps repeat 4 times – each time drawing 1 side of the square. Yes, you could easily copy and paste two little lines, but that becomes much harder to do in a large program.
Simplify the Code
So, let’s shorten the code:
repeat 4 times:
- Move the turtle forward by x pixels.
- Turn left.
As you can see, the amount of lines needed to draw the square has reduced without changing the output. It’s that easy!
KISS
One of the first acronyms I teach my students is KISS:

Graphics with Loops in Python Turtle
The for loop is Python’s counting loop. It can be used to keep track of the number of repetitions.
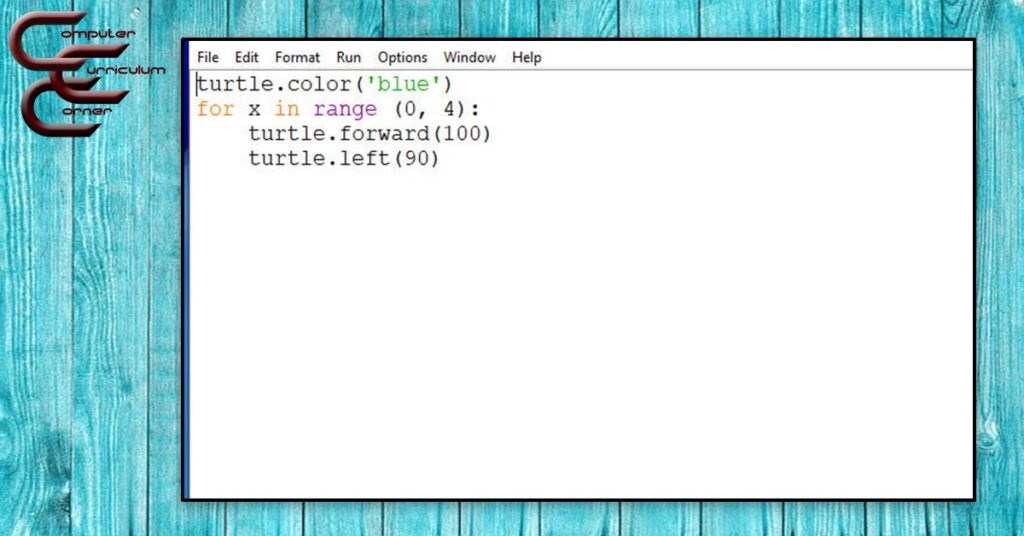
One way to find errors is by tracing the loop values to ensure that the logic is right:
for x in range (0, 4)
x | forward(x) |
0 | forward(100) |
1 | forward(100) |
2 | forward(100) |
3 | forward(100) |
4 | stop! |
The x variable counts from 0 to 4 by adding 1 each time the loop repeats. The loop stops when it reaches the last value.
Graphics with Loops in Python Turtle Tip!
Do the trace first before you code. That way, you can see how the values are changing, which will help you write the for loop range!
The Importance of Loops
A loop contains a sequence of events in a specific order. Understanding how a sequence works is an important step in coding so practicing can help a new coder. Share on X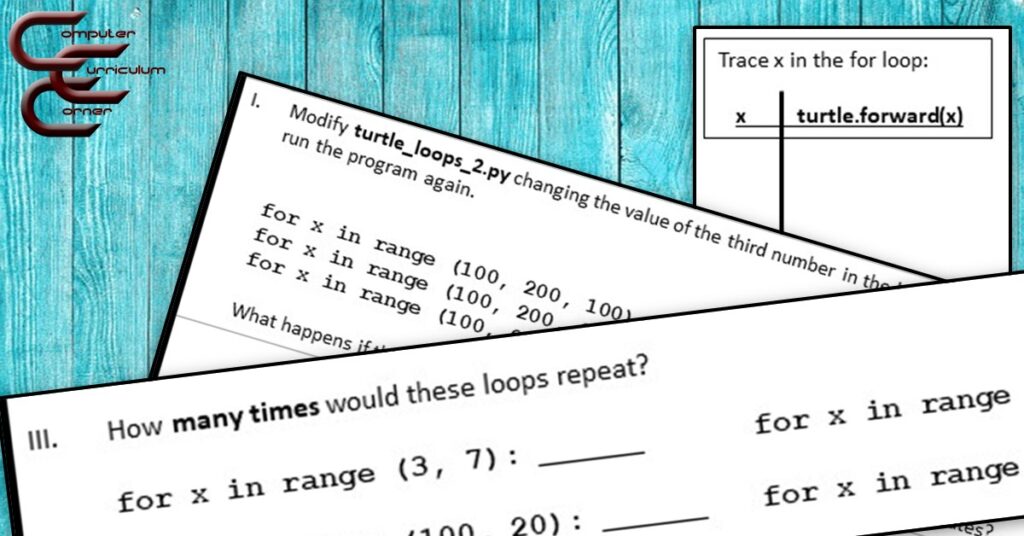
I have a resource if you’re interested in teaching turtle graphics with loops. It includes answer keys as well as lessons with teacher notes.
You can also buy the full unit (which includes two tests) here.
This unit includes Online Learning slides with embedded videos that can be used in Google Classroom on Microsoft One Drive, as well as a zipped PDF file with slides, answer keys, rubrics and code exemplars.
Basic Graphics Functions
If you haven’t used turtle’s graphics functions yet, hopefully, this post will help.
More Graphics with Loops in Python Turtle Ideas!
Looping lines can be fun, but find out what happens when you loop circles!