So, how do computers keep track of information? With a VARIABLE, of course! Almost all programs need variables to store data. The program may need to store the file name, date, sentences, math values, equations, or just about any piece of data that can change or vary. Hence, the name variable!
Why Do Programs Need Variables?
Computers actually store information differently depending on what type of data it is. It’s the programmer’s job to make sure that the contents of the variable match what the computer is expecting.
Now, Python is more forgiving than most languages. It lets you use variables almost any way you want. It does have some limits and rules (or syntax – yes, syntax! Just like English!), but you usually don’t have to tell the computer what type of info is being stored first.
When you use a variable in other languages, you have to explain to the computer what type of data you’re storing before you can use it. AND, you can’t change the data type once it’s been set.
So, if I want to store a math statement in Python, I just type: x = a + b
Seems simple, right?
Too simple!

Variables Have Types?
Programs need variables but computers still need to know what type of data you want to store. Think of a computer’s memory like your refrigerator. Every shelf has a purpose based on its difference height and size.
Take a look at the shelves in your fridge. They all vary in height. You can’t store a tall carton of milk, or a bottle of pop, on a small shelf. They only fit on shelves that have enough clearance or height.
Same with variables.
Each variable type tells the computer how much space or RAM it will need as well as how to store it.
Alpha vs Numeric
Numbers and letters aren’t stored the same way.
A number can be stored as a letter, but if you do that, the computer can’t do any math with it.
And, to make things more complicated, every programming language has at least two numeric types. Python has integer (int) and float (decimal) numbers.

Integer values take up less RAM than float, and they aren’t stored the same way. So, although you can store an int in a float, you can’t store a float in an int.
Then there’s text. Text is commonly called a string in programming.
A string consists of one or more characters.
Most languages use a single quotation to delineate a single character, and double quotes to indicate a string. Python is a very forgiving language. You can use either single or double quotations to indicate a string in Python.
When Does Python Need to Know the Variable Type?
Data types are relevant when you get input.
Python always assumes keyboard input is a string, meaning, printable keyboard characters. But, since you can’t do math with “45”, you have to tell Python that you want to store the input as either an int or float.
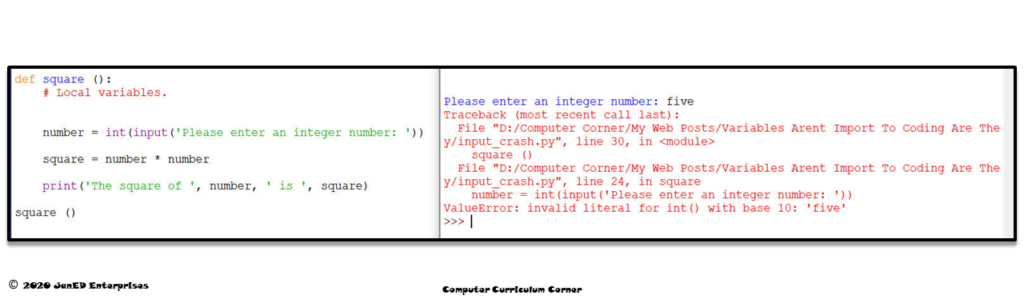
Ever Crashed A Program?
When a coding language tries to store a value that doesn’t match the type it’s expecting it’s going to go down in flames!
Coding languages don’t automatically check to see if the data and the type match. That’s the coder’s responsibility. So, remember to error trap your input or your program is going to unexpectedly go belly up if the variable and data don’t match.
Programs Need Variables To Save Memory
One of the biggest issues with today’s programs is that no one seems to care how much memory their code is going to use.
All variables take up memory. If the data is only needed for a short while or code segment, then it really doesn’t need to be “alive” throughout a program.

Global or Local?
Programs usually have two different ways to initialize or set up variables.
When a variable is used in a restricted setting like a structure or function, it’s called a local variable.
Variables that need to be used in multiple locations or structures are called global.
Usually, global variables are set up at the top of a program so they can be used anywhere.
Python isn’t like that.
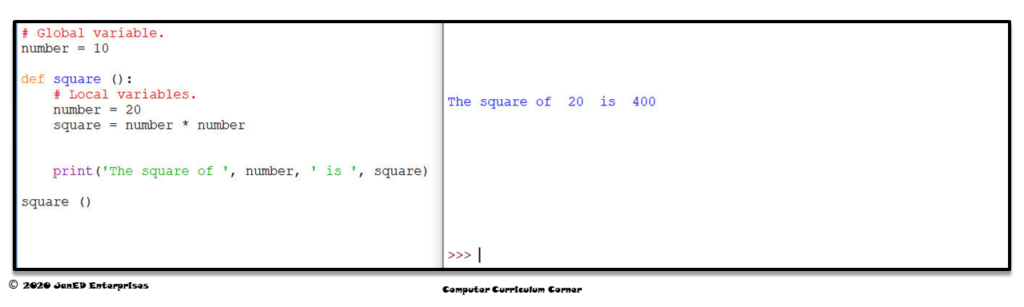
Python assumes that all variables are local UNLESS you tell it otherwise.
Every time…
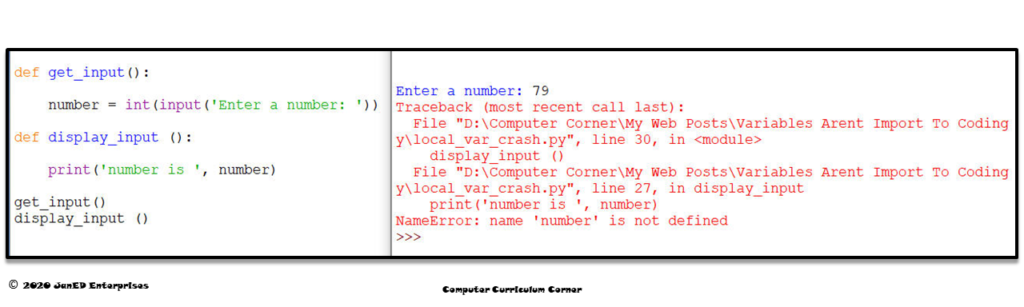
So, when you need to use a global variable, you have to put the keyword global in the statement before accessing the variable.
What’s in a Name?
Every language has its own conventions when it comes to naming things. But, they all pretty much agree on one thing: the name of something should reflect what it is doing/storing. Share on XCode tends to be thousands of lines, so accurately naming variables and structures can be very important. A variable’s name should be long enough that another coder knows its purpose.
Single letters are great for loop variables, but that’s all.
Python programmers use all lower case letters. Longer variable names with multiple words are natural. Words are joined by underscores to make it easier to read.
So, firstname becomes first_name in Python.
Java and C/C++ capitalize the first letter after the initial word: firstName, to make it easier to read.
The Bottom Line
Programs need variables. Every language has its own conventions or rules, so coders need to use variables and consistently use the naming conventions for the language they’re coding in.
More Variables!
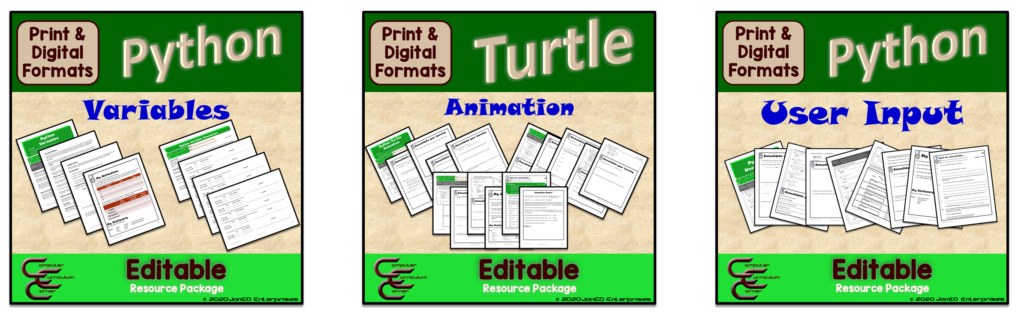