So, how do computers keep track of information? With a VARIABLE, of course! Almost every program needs variables to store data. The program may need to store the file name, date, sentences, math values, equations, or just about any piece of data that can change or vary. Hence, the name variable! But, Turing variables are extra special!
Why Does Turing Need Variables?
Computers actually store information differently depending on what type of data it is. It's the programmer's job to make sure that the contents of the variable match what the computer is expecting. Click To TweetNow, Turing is pretty strict. Using a variable is a two-step process. When you use a variable in most languages, you have to explain to the computer what type of data you’re storing before you can use it. AND, you can’t change the data type once it’s been defined.
Declaring A Turing Variable
First, you have to create a declaration statement. This statement tells Turing that you want to create a new variable by giving it a name and defining the type of data it will be storing.

var is Turing’s keyword to declare the variable. firstName is the name of the variable, and string, meaning text, is the type of data it will be storing.
Assigning a Turing Variable
The second step is to store data into the variable. There are two ways to do this: by getting user input or by assigning a specific value to the variable.
It’s obvious why you’d want to get user input in a program. But, why would you assign a value?
Say, your program calculates the square of a number. You’d need one value from the user: a number. You would then calculate the square of the number, which is a math statement: square = number x number.
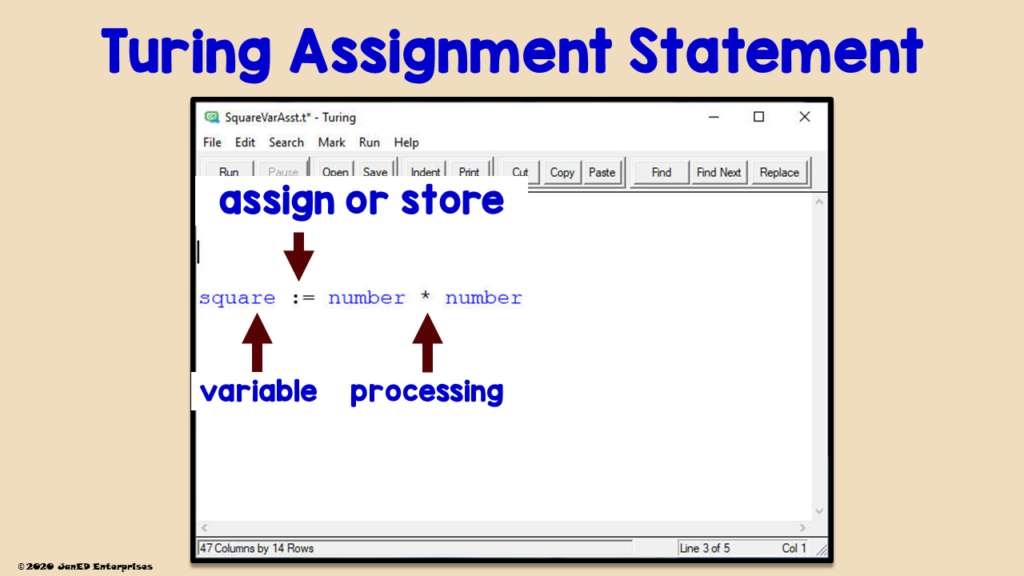
There’s your assignment statement: square := number * number.
Notice that the assignment operator is a colon with an equal sign.
Turing Variable Types
Computers need to know what type of data you want to store. Think of a computer’s memory like your refrigerator. Every shelf has a purpose based on its height and size.
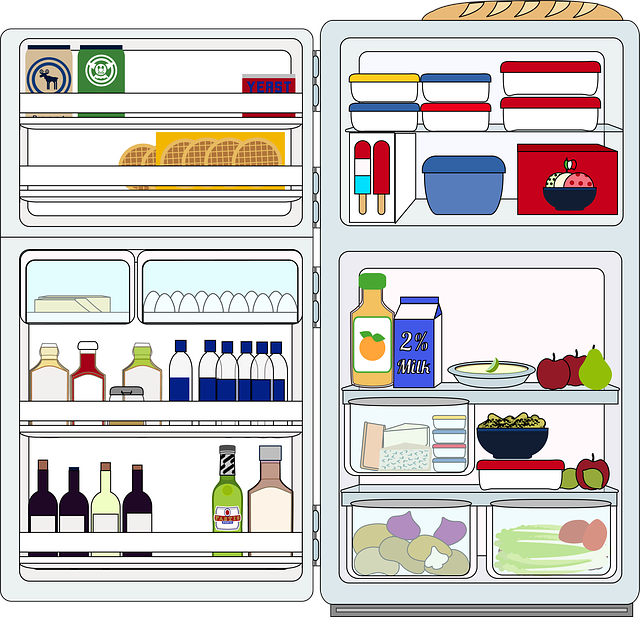
Take a look at the shelves in your fridge. They all vary in height. You can’t store a tall carton of milk, or a bottle of pop, on a small shelf.
It’s the same with variables.
Each variable type tells the computer how much space or RAM it will require as well as how to store it.
Alpha vs Numeric Characters
Numbers and letters aren’t stored the same way.
You can store a number as a letter. But if you do that, the computer use it in any math calculations.
And, to make things more complicated, every programming language has at least two numeric types. Turing’s two variable types are integer (int) and real (decimal) numbers.
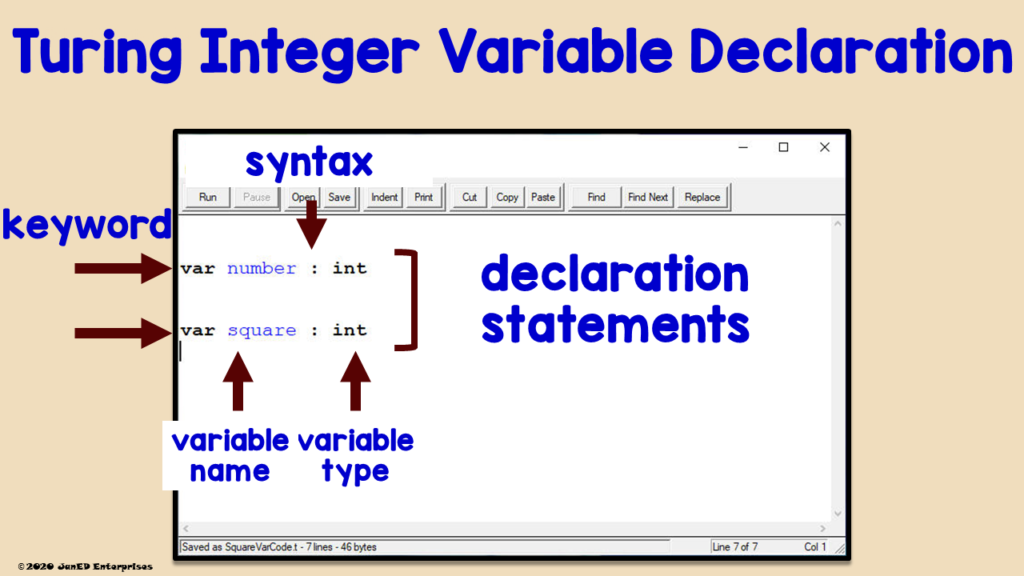
Integer values take up less RAM than real, and they aren’t stored the same way. So, although you can store an int in a real, you can’t store a real in an int.
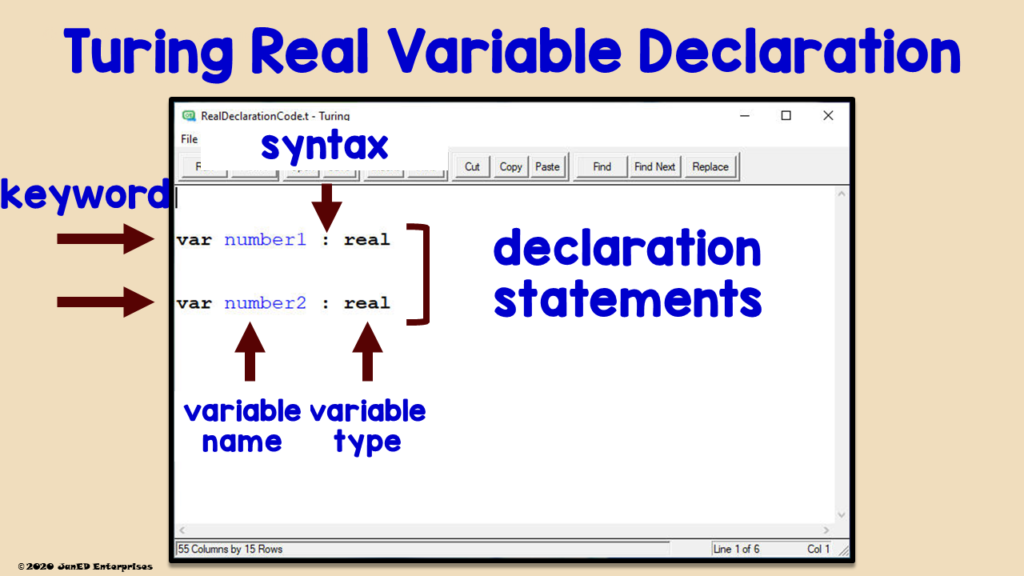
Then there’s text. Text is commonly called a “string” in programming.
A string consists of one or more characters.
Most languages, Turing included, use a single quotation to delineate a single character and double quotes to indicate a string.
String or Int?
Data types are relevant when you get input.
Turing requires all variables to be declared first, so it already knows what type of input will be stored. Just remember if a value is stored as a string, you can’t do any math calculations.
Crashing Programs Can Be Easy!
When a coding language tries to store a value that doesn’t match the variable type, your program is going to go down in flames!
Coding languages don't automatically check to see if the data and the type match. That's the coder's responsibility. Click To TweetSo, remember to error trap your input or your program is going to unexpectedly go belly up if the variable and data don’t match.
Saving Memory
One of the biggest issues with programs today is that no one seems to care how much memory their code is going to use.
All variables take up memory. If the data is only needed for a short while or code segment, then it really doesn’t need to be “alive” throughout a program.
Global or Local?
Programs usually have two different ways to initialize or set up variables.

When a variable is used in a restricted setting like a structure or procedure, it’s called a local variable.
Variables that need to be available in multiple locations or structures are called global.
Global variables are set up at the top of a program so they can be accessed anywhere.
What’s in a Name?
Every language has its own conventions when it comes to naming things. But, they all pretty much agree on one thing: the name of something should reflect what it is storing.
Code can be thousands of lines, so accurately naming variables and structures can be very important. A variable’s name should be long enough that another coder knows its purpose.
Single letters are great for loop control variables, but that’s all.
Turing is very forgiving. As long as the variable name is alpha-numeric or contains specific punctuation characters like “.” (period) or “_” (underscore), it will work.
This versatility is one reason Turing is an excellent beginner language.
You can use it to teach the naming conventions from another language, so kids get used to them. Then, when they move on to a more complex language like Java or Python, they already know the naming conventions.
Say you want to store the user’s first name. The variable’s name should reflect what it will store, so it should be called “first name”.
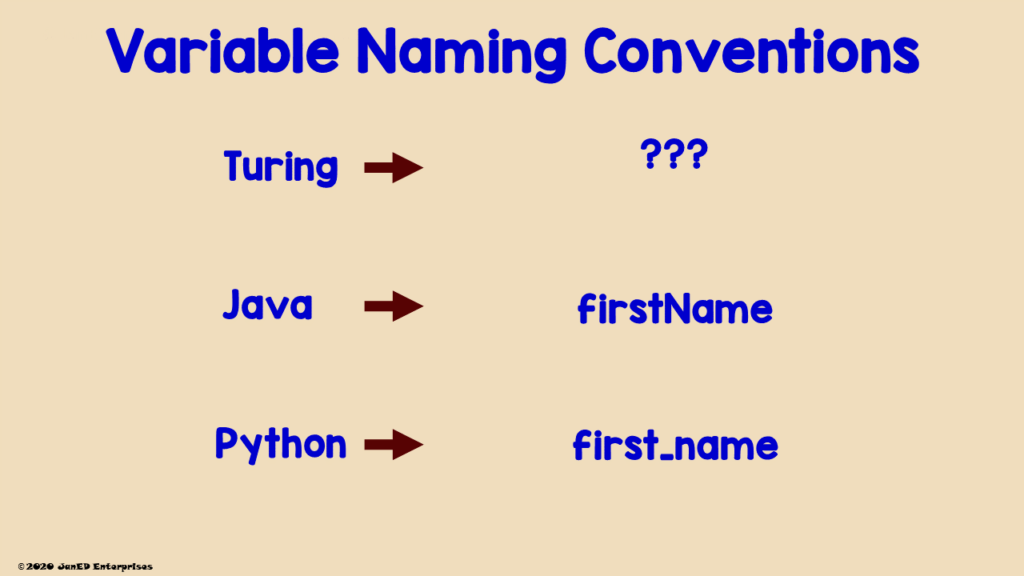
Since blank spaces can’t be used in variable names, Java and C/C++ capitalize the first letter after the initial word firstName to make it easier to read. This technique is known as the camel approach.
Whereas, the variable name would be first_name in Python. For more information about Python and its variable types click here.
My Turing assignments use the Java and C/C++ naming conventions.
The Bottom Line
Programs use variables all the time.
Coders need to know the precise syntax required to use variables as well as following the naming conventions for that specific language. Click To TweetSo, don’t dismiss Turing as a possible beginner language!
Resources About Variables!
If you’re interested in learning more about variables, check out these resources for Turing and Python.